Middleware in ASP.NET Core
.NET CORE provides you flexibility to compose your request pipeline using middleware. .NET Core middleeare performs asynchronous logics on an HTTPContext and then either invokes the next middleware in the squence or terminates the request directly.
A middleware content called Mvc is added by invoking an UseMvc() extension method in the configuration method.
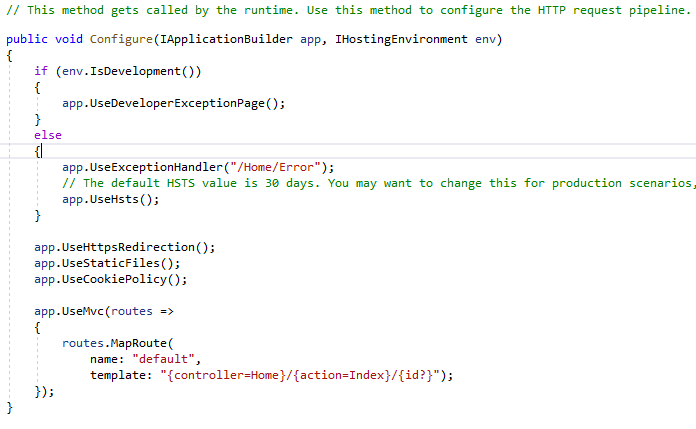
In Configure() method, IApplicationBuilder interface is used to configure the requrest pipeline.
The sequesce of middilewares matters.
Configure middleware using Use, Run and Map
You can configure a middleware by using Use, Run and Map
Used for invoking next midleware if present. By using Use, middleware execute and pass control to next middleware if present in request pipeline.
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app)
{
app.Use(async(context, next) =>
{
awaitcontext.Response.WriteAsync("Use- 1st middileware executed; ");
awaitnext.Invoke();
});
app.Use(async(context, next) =>
{
awaitcontext.Response.WriteAsync("Use- 2nd middileware executed;");
awaitnext.Invoke();
});
}

Used for middleware that will terminate the request and wont allow to invoke next middleware in pipeline. It recommanded to use Run in last of pipeline.
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app)
{
app.Use(async(context, next) =>
{
awaitcontext.Response.WriteAsync("Use- 1st middileware executed; ");
awaitnext.Invoke();
});
app.Run(asynccontext =>
{
awaitcontext.Response.WriteAsync("Run- 2nd middileware executed; ");
});
app.Use(async(context, next) =>
{
awaitcontext.Response.WriteAsync("Use- 3rd middileware executed;");
awaitnext.Invoke();
});
}

Used for branching the pipeline. Map accept path as first parameter and delegate function as second parameter. If given path matched then execute the branch.
MapWhen - Used to branches the request pipeline based on the result of the given predicate
public void Configure(IApplicationBuilder app)
{
app.Use(async(context, next) =>
{
awaitcontext.Response.WriteAsync("Use- middileware executed; ");
awaitnext.Invoke();
});
app.Map("/MapRequest", MapRquestTesting);
}
private static void MapRquestTesting(IApplicationBuilder app)
{
app.Run(asynccontext =>
{
awaitcontext.Response.WriteAsync("Map- middileware executed;");
});
}


UseDeveloperExceptionPage - Used for redirecting on developer exception page(built-in) error page if exception occurred
UseDatabaseErrorPage
UseMvc - Use for adding MVC to request pipeline and handling mvc requests
UseExceptionHandler - Used for handling exception
CORS - Used for Cross-Origin Resource Sharing
UseHttpsRedirection - Used for redirecting all http request to https
UseStaticFiles - Used for serving static files and directory browsing
UseCookiePolicy - Used for tracks consent from users for storing personal information
UseAuthentication - Used for authentication perpose
UseSession - Used for storing data in session
UseRouting - Used for defining route constraint